Project: Wone
The Wone project involved designing a wearable drone that can fly around the user for aerial cinematography. This innovative drone was built using custom toroidal propellers, gyroscopic sensors, and lightweight batteries to ensure a compact yet slightly functional prototype. The design allowed users to easily capture panoramic videos of themselves or their surroundings from a drone POV, all without needing to carry bulky equipment. The project was pitched to investors during the Bath Hack 2023 competition in a Dragon’s Den-style business presentation.
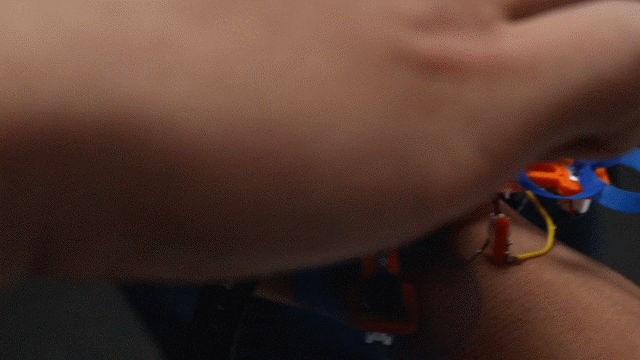
Project Description
As the electrical engineer for the Wone project, I was responsible for the complete design and implementation of the drone's embedded systems. Utilising an Arduino Nano, I developed custom code to integrate on-board accelerometers for stabilisation during flight, ensuring smooth operation and precise control. A key feature of the Wone was its use of toroidal propellers, which provided enhanced safety, reduced noise, and improved efficiency.
The project utilised 3D printing for rapid prototyping and lightweight lithium batteries to maintain portability and ensure a long flight time. The final product combined the convenience of a wristwatch with the functionality of a flying drone, allowing users to record stunning aerial footage with ease. The design was pitched to potential investors, along with a comprehensive 5-year business plan, cost analysis, and scalability forecasts. The Wone prototype demonstrated the potential for wearable drones in consumer markets, making it a unique solution for hands-free cinematography.
Key Features
- Wearable Drone Design: A wristwatch form factor combined with drone functionality, allowing users to capture aerial footage effortlessly.
- Custom Toroidal Propellers: Used to ensure safe operation around people and animals, while also reducing the noise output for a more pleasant user experience.
- On-Board Accelerometers: Provided real-time stabilisation during flight, ensuring smooth and accurate control of the drone’s movements.
- Arduino Nano Control: Custom programming on the Arduino Nano enabled seamless control of the motors and sensors, while optimising power usage.
- 3D Printing & Rapid Prototyping: Enabled the design and iteration of lightweight, functional components, essential for the compact nature of the wearable drone.
- Investors Pitch: A detailed 5-year business plan and cost forecast was prepared and presented to investors in a Dragon's Den-style competition, showcasing the potential market for wearable drones.
Technologies Used
The following technologies and tools were used in the development of the Wone wearable drone:
- Arduino Nano: Provided the processing power for motor control, stabilisation, and data gathering from sensors.
- C++: Custom code was written in C++ to control the drone’s flight and stabilisation systems, leveraging real-time sensor data.
- Gyroscopic Sensors & Accelerometers: Used for drone stabilisation and orientation control during flight.
- 3D Printing: Enabled rapid prototyping of drone components, ensuring a lightweight and durable structure.
- Rapid Prototyping: Allowed for the quick iteration and testing of designs to ensure both mechanical and electronic components functioned correctly.
- Cost Forecast & Business Plan: Developed a comprehensive 5-year business model, including cost analysis and scalability options for the Wone drone.
Project Gallery
Here are some images showcasing the Wone drone and its development process:
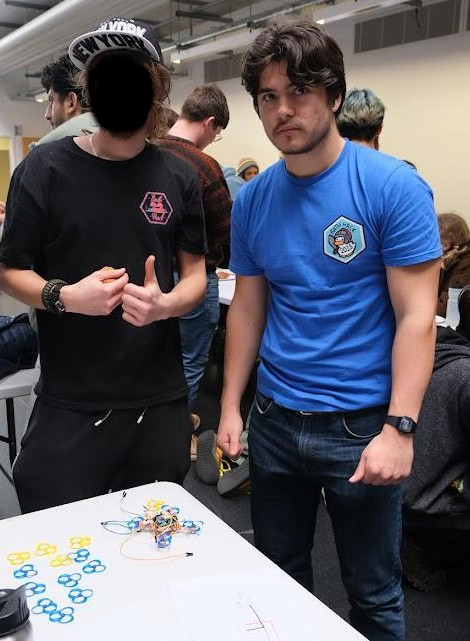
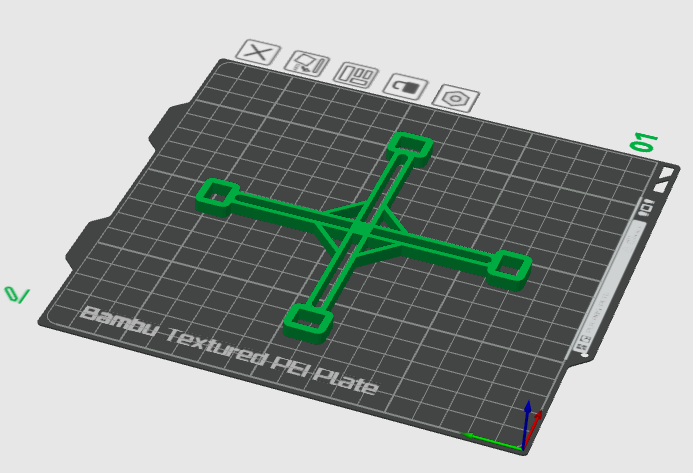
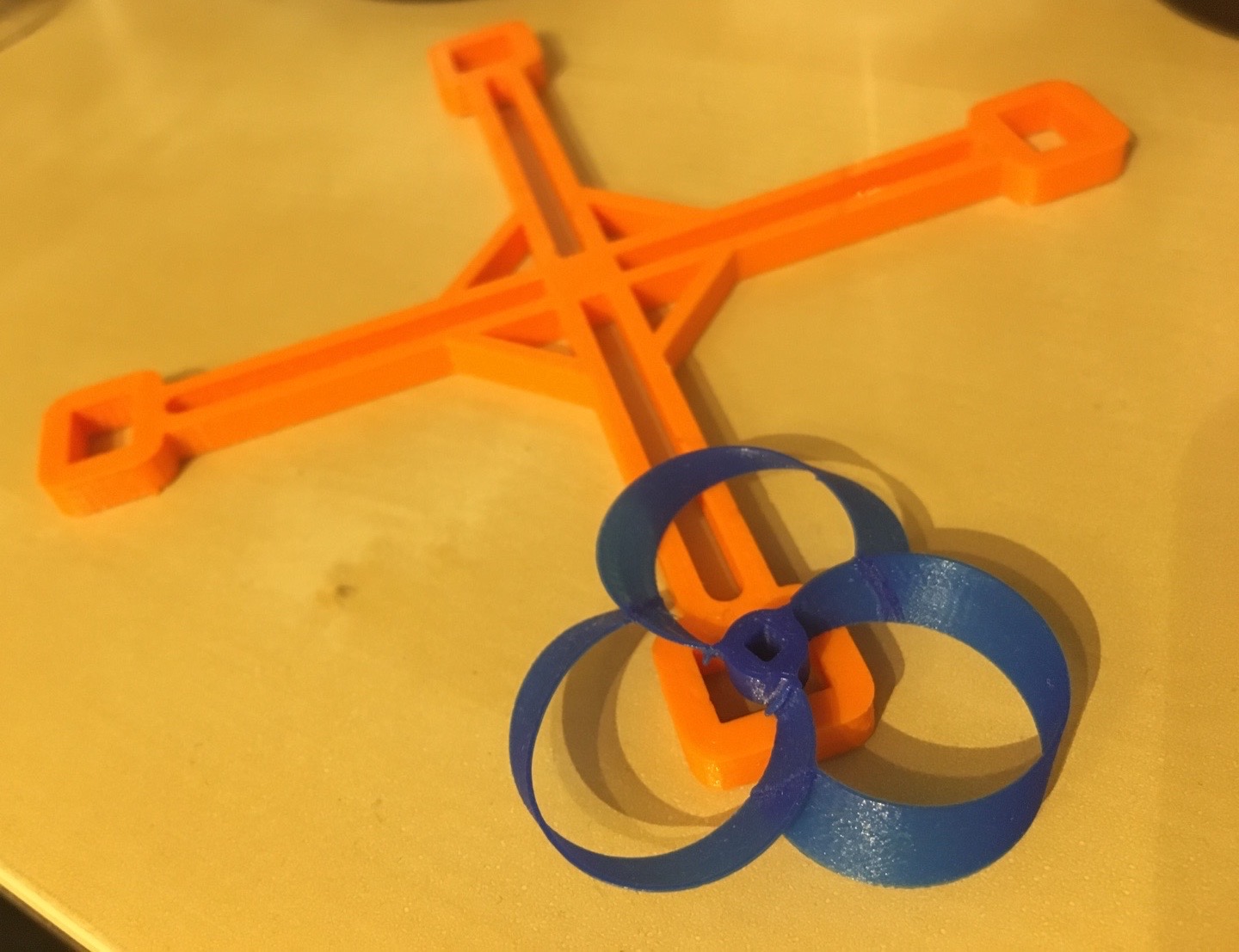
Project Demonstration
Watch the video below for a short pitch of the Wone drone:
Here is the code we developed:
#include <Wire.h>
#include <Adafruit_MMA8451.h>
#include <Adafruit_Sensor.h>
#define FL_MOTOR 3
#define FR_MOTOR 9
#define BR_MOTOR 10
#define BL_MOTOR 11
Adafruit_MMA8451 mma = Adafruit_MMA8451();
void setup(void) {
Serial.begin(9600);
if (! mma.begin()) {
Serial.println("Couldnt start");
while (1);
}
pinMode(FL_MOTOR, OUTPUT);
//pinMode(FR_MOTOR, OUTPUT);
//pinMode(BR_MOTOR, OUTPUT);
//pinMode(BL_MOTOR, OUTPUT);
mma.setRange(MMA8451_RANGE_2_G);
}
void loop() {
// Read the 'raw' data in 14-bit counts
mma.read();
Serial.print("X:\t"); Serial.print(mma.x);
Serial.print("\tY:\t"); Serial.print(mma.y);
Serial.print("\tZ:\t"); Serial.print(mma.z);
Serial.println();
/* Get a new sensor event */
sensors_event_t event;
mma.getEvent(&event);
analogWrite(FL_MOTOR, map(mma.x, -4000, 4000, 0, 256));
analogWrite(FR_MOTOR, map(mma.y, -4000, 4000, 0, 256));
analogWrite(BR_MOTOR, map(mma.y, -4000, 4000, 256, 0));
analogWrite(BL_MOTOR, map(mma.x, -4000, 4000, 256, 0));
/* Display the results (acceleration is measured in m/s^2)
Serial.print("X: \t"); Serial.print(event.acceleration.x); Serial.print("\t");
Serial.print("Y: \t"); Serial.print(event.acceleration.y); Serial.print("\t");
Serial.print("Z: \t"); Serial.print(event.acceleration.z); Serial.print("\t");
Serial.println("m/s^2 "); */
/* Get the orientation of the sensor */
//uint8_t o = mma.getOrientation();
delay(5);
}